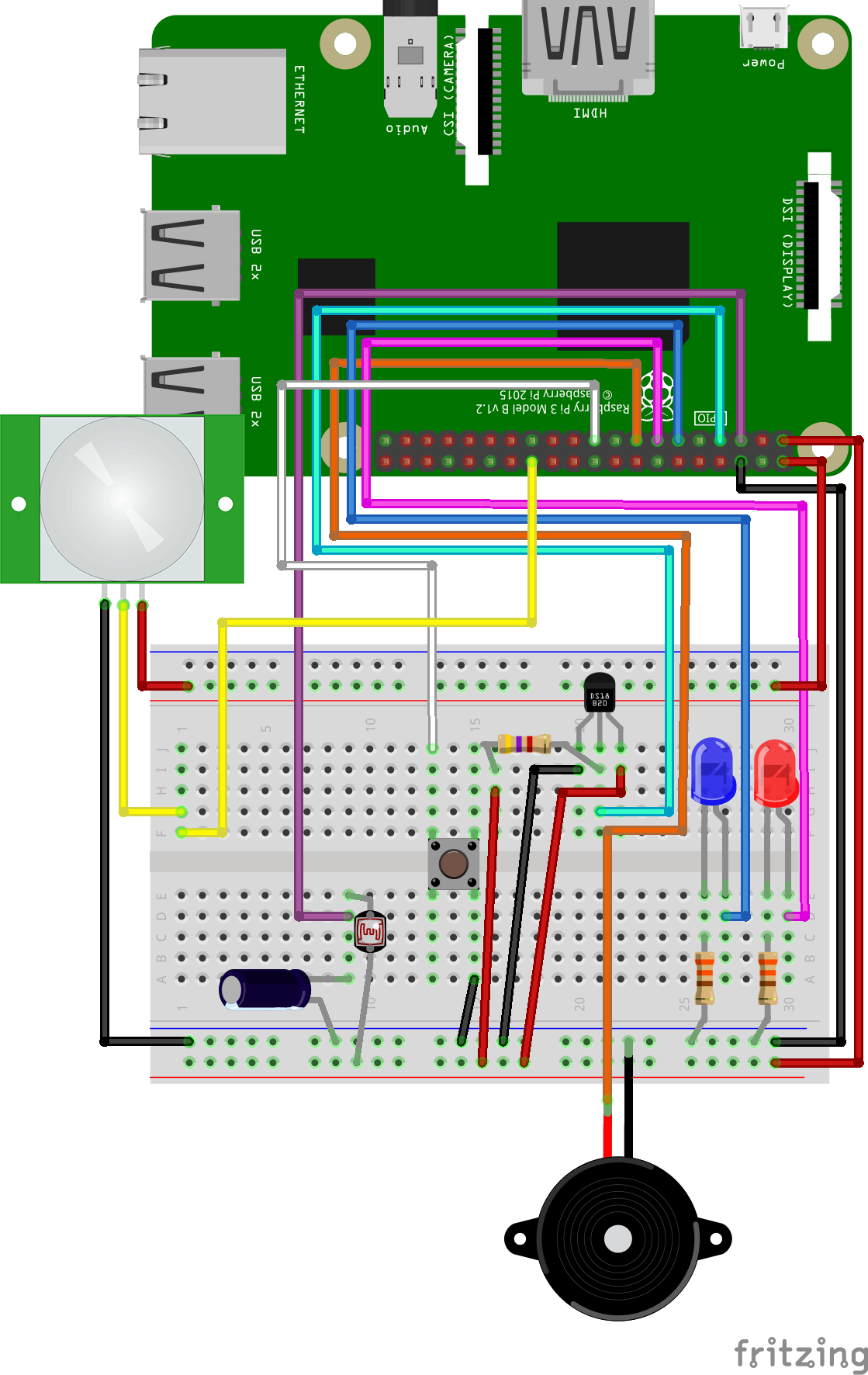
GPIO and Python (9/9) - PIR
In this project you will learn how to wire and program a passive infrared sensor.
Things you will need:
Raspberry Pi + SD Card
Keyboard + Mouse
Monitor + HDMI Cable
Power Supply
Breadboard
1x Red LED
1x Blue LED
2x 330? Resistor
5x M/M Jumper Wire
12x M/F Jumper Wire
1x Button
1x Buzzer
1x DS18B20 Temperature Sensor
1x 4k7? Resistor
1x 1uF Capacitor
1x Light Dependent Resistor (LDR)
1x PIR Sensor
Prerequisites:
Latest version of Rasbian installed on your SD Card
Raspberry Pi setup with a keyboard, mouse and monitor
1. Change the current directory to our gpio_python_code directory:
cd gpio_python_code
2. Start by creating a file for our pir script
touch 9_pir.py
3. Edit the 9_pir.py script using nano 9_pir.py add the following code:
#!/usr/bin/python import RPi.GPIO as GPIO from time import sleep GPIO.setmode(GPIO.BCM) GPIO.setup(27,GPIO.OUT) GPIO_PIR = 7 print "PIR Module Test (CTRL-C to exit)" # Set pin as input GPIO.setup(GPIO_PIR,GPIO.IN) Current_State = 0 Previous_State = 0 try: print "Waiting for PIR to settle ..." # Loop until PIR output is 0 while GPIO.input(GPIO_PIR)==1: Current_State = 0 print " Ready" # Loop until users quits with CTRL-C while True : # Read PIR state Current_State = GPIO.input(GPIO_PIR) if Current_State==1 and Previous_State==0: # PIR is triggered print " Motion detected!" # Record previous state GPIO.output(27,GPIO.HIGH) sleep(1) GPIO.output(27,GPIO.LOW) Previous_State=1 elif Current_State==0 and Previous_State==1: # PIR has returned to ready state print " Ready" Previous_State=0 # Wait for 10 milliseconds sleep(0.01) # clean up gpio pins when we exit the script except KeyboardInterrupt: print " Quit" # Reset GPIO settings GPIO.cleanup()
4. Execute your 9_pir.py script
sudo python 9_pir.py
5. Press ctrl+c to exit the script