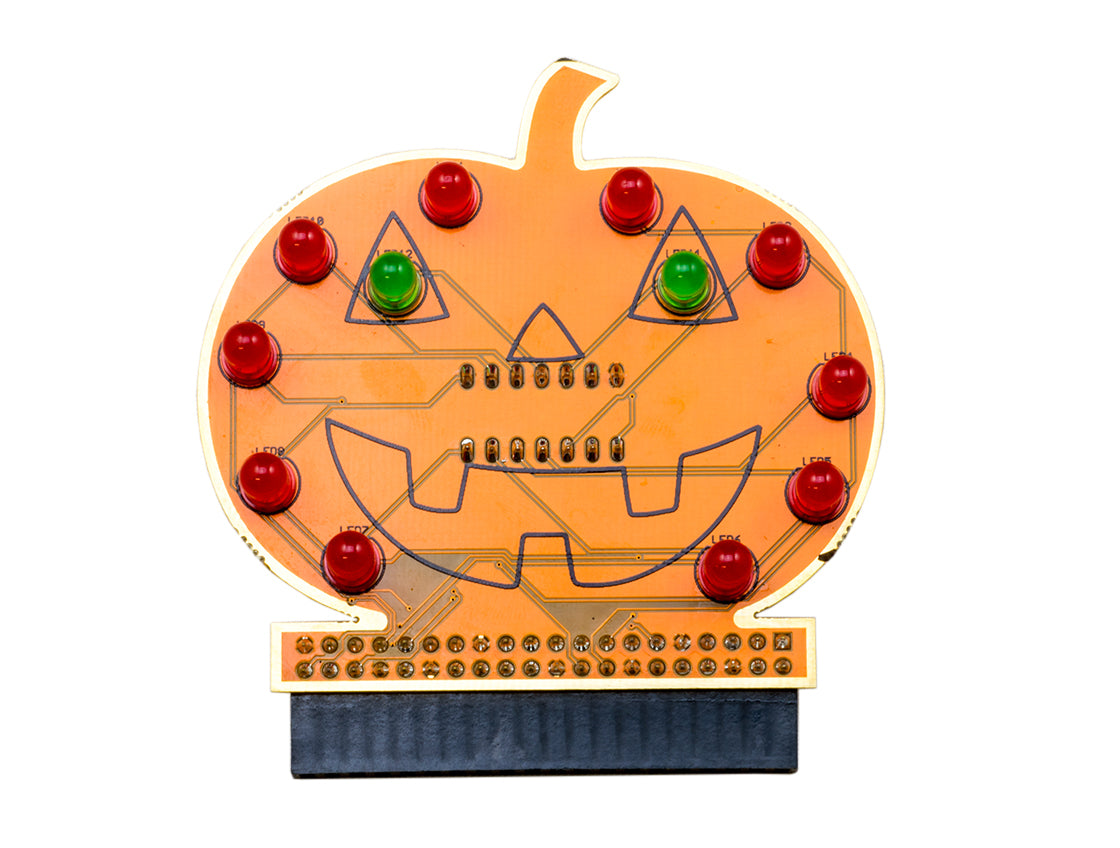
Using GPIO Zero with the PumpkinPi
It's that time of year... the ghosts and ghouls are out in force, pumpkins are being carved and witches are preparing their broomsticks, it's Halloween! And to celebrate we've got this tutorial on how to get started using GPIO Zero and our new PumpkinPi Programmable board. Lets get down and spooky with our Raspberry Pi...
Once you've got your board built and attached onto the Raspberry Pi, open a terminal and type:
git clone https://github.com/modmypi/Programmable-Pumpkin
This will download the Pumpkin Pi library. Inside pumpkinpi.py there is an extension to the GPIO Zero library which allows you to use GPIO Zero commands with the PumpkinPi.
Using File Manager, navigate into the Programmable-Pumpkin folder and start an IDLE session.
Firstly we need to import the PumpkinPi class into IDLE shell:
from pumpkinpi import PumpkinPi
And make a PumpkinPi object to use in Python:
pumpkin = PumpkinPi()
Now we can turn on all of the lights of the PumpkinPi by using:
pumpkin.on()
And we can turn off the pumpkin by using
pumpkin.off()
Each of the LEDs on the PumpkinPi has a name and can be individually turned on for example, if we want to turn on the left eye:
pumpkin.eyes.left.on()
The LEDs are labelled in the following way:
So you can turn on the eyes, each side and each LED by using it's name.
If you wanted to turn on the sides of the pumpkin we can use:
pumpkin.sides.on()
Or specifically the middle LEDs:
pumpkin.off() # To clear the lights so we can see what we're doing.
pumpkin.sides.left.middle.on()
pumpkin.sides.right.middle.on()
Once we've turned on some LEDs, we can now put some patterns in a loop to always play.
while True:
pumpkin.sides.left.on()
pumpkin.eyes.right.on()
sleep(.5)
pumpkin.off()
pumpkin.sides.right.on()
pumpkin.eyes.left.on()
sleep(.5)
pumpkin.off()
This will make the left side and right eye come on, then it'll swap to the other side and other eye until you hit CTRL C to finish.
Instead of this long loop of on off commands, we can use the toggle command
pumpkin.off() # Just to make sure our pumpkin starts off.
pumpkin.sides.left.on()
pumpkin.eyes.right.on()
while True:
pumpkin.toggle()
sleep(.5)
This will do the same as the first long while loop we used, except we don't need the off statements or the extra on statements.
As well as toggle, there is blink, try typing:
pumpkin.blink()
And your pumpkin will blink away at you.
We can change the speed of the blinking by adding a few parameters:
pumpkin.blink(2,.5)
This will make your pumpkin blink for on for 2 seconds and off for half a second.
When you're finished making your eyes blink, don't forget to use a close |command to close the GPIO pins off.
pumpkin.close()
As well as on, off, blink and toggle, we can make our Pumpkin glow with a spooky PWM (pulse width modulation) setup.
Now we've closed our pumpkin, we need a new one, so lets make one using the following command:
pumpkin = PumpkinPi(pwm=True)
All of the on, off, toggle and blink commands will still work, but now we get the extra pulse command.
pumpkin.pulse()
Which makes our pumpkin fade in and fade out at a given pace.
Lets bring it all together a create a script which can be run all the time using some of the PWM functions, referencing LEDs and some of the normal on/off functions
Open a new file called spooky_display.py and add the following code:
from pumpkinpi import PumpkinPi
from time import sleep
# Define a function to control our Pumpkin which takes a PumpkinPi as an argument.
# This script contains flashing LEDs, please take care if you are sensitive to flashing lights.
def pump_pulse(pumpkin):
pumpkin.sides.left.bottom.pulse(5, 0.5, 1) # Fade in for 5 seconds, fade out for .5 seconds and do this once.
pumpkin.sides.right.bottom.pulse(5, 0.5, 1)
sleep(.5)
pumpkin.sides.left.midbottom.pulse(4.5, 0.5, 1)
pumpkin.sides.right.midbottom.pulse(4.5, 0.5, 1)
sleep(.5)
pumpkin.sides.left.middle.pulse(4, 0.5, 1)
pumpkin.sides.right.middle.pulse(4, 0.5, 1)
sleep(.5)
pumpkin.sides.left.midtop.pulse(3.5, 0.5, 1)
pumpkin.sides.right.midtop.pulse(3.5, 0.5, 1)
sleep(.5)
pumpkin.sides.left.top.pulse(3, 0.5, 1)
pumpkin.sides.right.top.pulse(3, 0.5, 1)
sleep(.5)
pumpkin.eyes.blink(.1, .1, 0, 0, 12) # Blink on for .1 seconds, off for .1 seconds, do not fade and do this 12
sleep(3)
pumpkin.off()
pumpkin = PumpkinPi(pwm=True)
while True:
pump_pulse(pumpkin)
else:
pumpkin.close()
Now hit F5 inside IDLE to boot the script and watch your pumpkin scare your friends and family...
For further projects using GPIO Zero, have a look at the Raspberry Pi Foundation's free Simple Electronics with GPIO Zero book which can be downloaded from their website.
If you've got any frightening scripts to share using the PumpkinPi, make sure you share them in the comments below.